How Builders Can Strengthen Their Debugging Expertise By Gustavo Woltmann
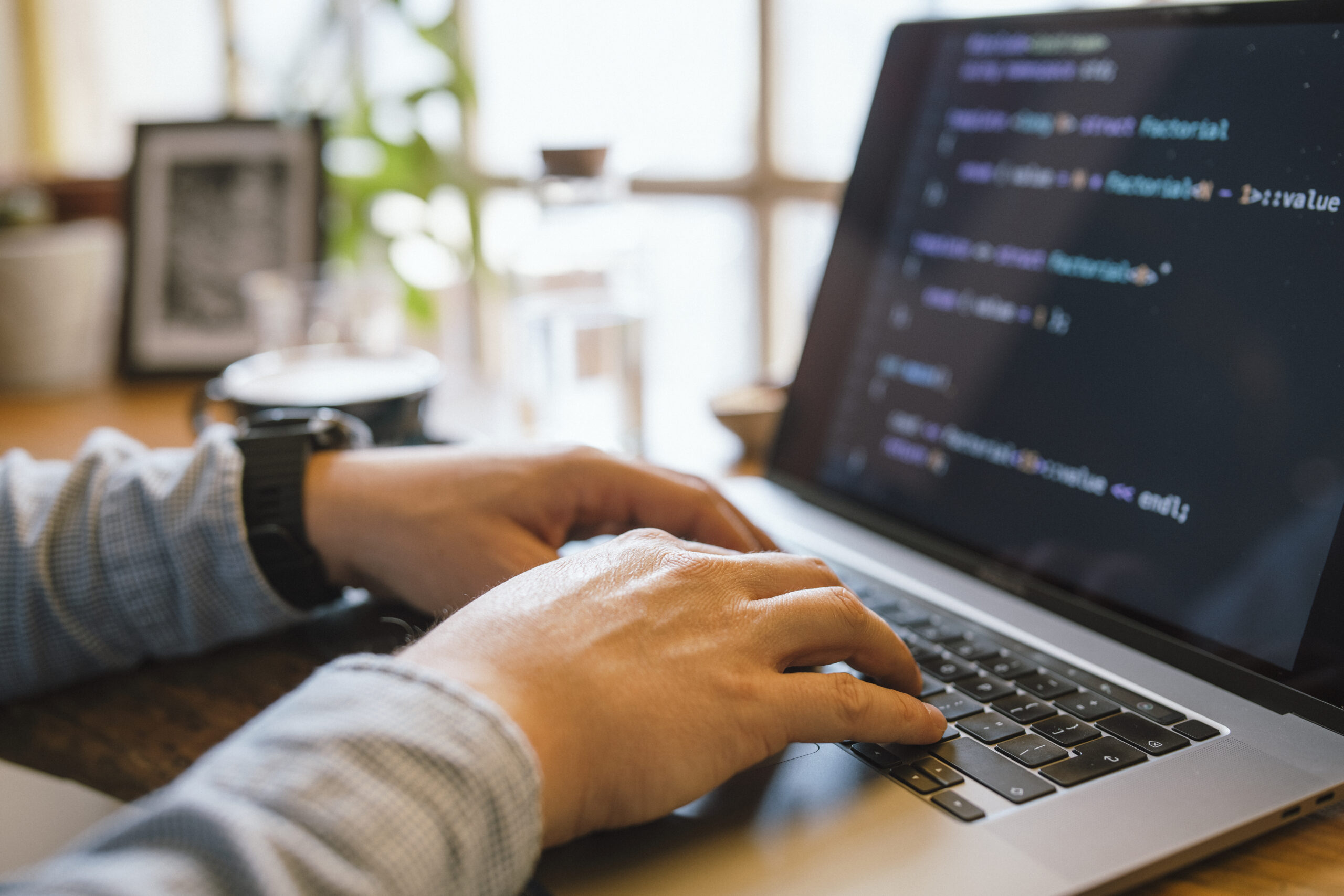
Debugging is Just about the most necessary — however usually neglected — competencies in a developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about comprehending how and why items go Improper, and Discovering to think methodically to solve problems effectively. Regardless of whether you're a newbie or maybe a seasoned developer, sharpening your debugging competencies can help you save several hours of annoyance and radically help your productivity. Listed here are a number of strategies to help builders stage up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods developers can elevate their debugging abilities is by mastering the tools they use everyday. Though producing code is a single A part of advancement, understanding how to connect with it properly in the course of execution is equally significant. Fashionable growth environments arrive Geared up with strong debugging capabilities — but lots of builders only scratch the surface area of what these tools can perform.
Consider, such as, an Integrated Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources allow you to established breakpoints, inspect the worth of variables at runtime, phase by way of code line by line, as well as modify code over the fly. When employed correctly, they Enable you to notice accurately how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-finish builders. They allow you to inspect the DOM, keep an eye on community requests, see authentic-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into manageable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate above jogging processes and memory management. Finding out these applications may have a steeper Understanding curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become comfy with Edition Regulate units like Git to grasp code heritage, obtain the exact moment bugs had been introduced, and isolate problematic variations.
Ultimately, mastering your tools indicates heading over and above default options and shortcuts — it’s about establishing an personal knowledge of your development atmosphere in order that when concerns come up, you’re not dropped at the hours of darkness. The greater you are aware of your applications, the greater time you may expend resolving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and infrequently missed — techniques in productive debugging is reproducing the challenge. Just before jumping into the code or creating guesses, builders have to have to make a steady atmosphere or scenario exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a game of probability, typically leading to wasted time and fragile code variations.
Step one in reproducing an issue is accumulating just as much context as you possibly can. Talk to questions like: What steps led to The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The more element you've got, the easier it will become to isolate the exact disorders beneath which the bug occurs.
As you’ve collected more than enough data, try to recreate the situation in your local environment. This might necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If the issue appears intermittently, take into consideration creating automatic tests that replicate the edge scenarios or state transitions associated. These tests not merely assistance expose the issue and also prevent regressions Later on.
Sometimes, The problem can be environment-certain — it would happen only on particular running methods, browsers, or beneath unique configurations. Applying resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms may be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t simply a step — it’s a attitude. It calls for tolerance, observation, in addition to a methodical strategy. But as soon as you can continually recreate the bug, you might be already halfway to fixing it. With a reproducible circumstance, You should utilize your debugging applications extra correctly, exam potential fixes properly, and connect a lot more Obviously along with your group or consumers. It turns an abstract complaint right into a concrete obstacle — and that’s where developers prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most worthy clues a developer has when anything goes Mistaken. As opposed to viewing them as irritating interruptions, builders really should learn to take care of mistake messages as direct communications from the procedure. They generally inform you just what occurred, exactly where it happened, and from time to time even why it took place — if you know how to interpret them.
Get started by looking at the concept meticulously and in comprehensive. A lot of developers, specially when beneath time pressure, look at the 1st line and right away begin earning assumptions. But deeper in the mistake stack or logs may lie the genuine root bring about. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them very first.
Crack the error down into pieces. Can it be a syntax error, a runtime exception, or possibly a logic error? Does it issue to a particular file and line selection? What module or operate triggered it? These issues can manual your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Mastering to acknowledge these can dramatically hasten your debugging approach.
Some faults are vague or generic, and in All those cases, it’s important to look at the context wherein the mistake occurred. Check out associated log entries, input values, and up to date adjustments during the codebase.
Don’t overlook compiler or linter warnings both. These usually precede larger sized problems and provide hints about probable bugs.
Ultimately, error messages will not be your enemies—they’re your guides. Discovering to interpret them effectively turns chaos into clarity, helping you pinpoint problems more rapidly, decrease debugging time, and become a far more successful and self-confident developer.
Use Logging Sensibly
Logging is The most highly effective tools inside a developer’s debugging toolkit. When utilized efficiently, it provides actual-time insights into how an application behaves, serving to you have an understanding of what’s happening under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic facts for the duration of growth, Data for common occasions (like successful get started-ups), Alert for likely difficulties that don’t split the application, Mistake for genuine troubles, and FATAL when the process can’t keep on.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your method. Focus on critical functions, condition modifications, enter/output values, and significant choice details within your code.
Format your log messages Plainly and regularly. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in generation environments exactly where stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. Which has a nicely-assumed-out logging strategy, you may reduce the read more time it requires to identify issues, obtain further visibility into your purposes, and Enhance the Over-all maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not merely a technical activity—it is a method of investigation. To properly establish and fix bugs, developers need to technique the procedure similar to a detective solving a mystery. This attitude helps break down complicated concerns into workable sections and abide by clues logically to uncover the foundation cause.
Start by gathering proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, collect as much pertinent details as it is possible to with no leaping to conclusions. Use logs, examination situations, and consumer studies to piece collectively a clear picture of what’s taking place.
Subsequent, type hypotheses. Ask yourself: What could be producing this actions? Have any improvements not long ago been manufactured to your codebase? Has this challenge transpired just before under similar instances? The target is usually to narrow down possibilities and determine potential culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge inside a managed natural environment. Should you suspect a specific purpose or element, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcomes direct you nearer to the truth.
Spend shut notice to modest particulars. Bugs normally conceal in the minimum expected destinations—like a lacking semicolon, an off-by-1 error, or possibly a race situation. Be extensive and affected person, resisting the urge to patch The problem with out thoroughly comprehending it. Momentary fixes might cover the true trouble, only for it to resurface later on.
Last of all, hold notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging approach can save time for long run issues and support others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more effective at uncovering hidden troubles in elaborate systems.
Compose Assessments
Producing checks is one of the most effective approaches to transform your debugging skills and All round progress performance. Checks not only aid catch bugs early and also function a security Web that offers you self-confidence when producing alterations on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem happens.
Begin with unit tests, which give attention to personal features or modules. These tiny, isolated exams can rapidly reveal whether or not a specific piece of logic is Operating as expected. When a exam fails, you straight away know where by to glance, appreciably minimizing time invested debugging. Device tests are Primarily practical for catching regression bugs—difficulties that reappear soon after Formerly becoming preset.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These support make certain that various aspects of your application function alongside one another efficiently. They’re specifically useful for catching bugs that manifest in intricate methods with numerous factors or providers interacting. If something breaks, your assessments can let you know which Element of the pipeline failed and less than what problems.
Creating assessments also forces you to Assume critically about your code. To test a attribute correctly, you would like to grasp its inputs, expected outputs, and edge scenarios. This degree of understanding In a natural way leads to higher code composition and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails regularly, you may focus on repairing the bug and enjoy your test go when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Later on.
Briefly, writing exams turns debugging from a discouraging guessing game into a structured and predictable method—helping you catch a lot more bugs, speedier plus more reliably.
Consider Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the condition—staring at your display for hrs, making an attempt solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks allows you reset your intellect, cut down frustration, and often see the issue from the new standpoint.
When you're as well close to the code for too lengthy, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain will become a lot less productive at dilemma-fixing. A short wander, a espresso split, or perhaps switching to a different task for ten–15 minutes can refresh your target. Numerous developers report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done inside the background.
Breaks also help reduce burnout, Specially in the course of lengthier debugging classes. Sitting down in front of a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed energy in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
Should you’re trapped, an excellent general guideline is usually to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It may well come to feel counterintuitive, especially beneath limited deadlines, nevertheless it basically contributes to a lot quicker and more effective debugging In the long term.
In brief, getting breaks is not a sign of weak point—it’s a sensible tactic. It gives your brain Place to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each one can educate you anything important if you take some time to replicate and review what went Incorrect.
Commence by asking by yourself some critical thoughts as soon as the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The solutions typically expose blind spots within your workflow or comprehension and allow you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great behavior. Maintain a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Anything you figured out. After some time, you’ll begin to see patterns—recurring problems or common issues—you could proactively prevent.
In crew environments, sharing Everything you've discovered from the bug with the peers can be Primarily highly effective. No matter whether it’s through a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar situation boosts group performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as crucial parts of your growth journey. In the end, a lot of the greatest builders usually are not those who create fantastic code, but people who consistently find out from their issues.
Ultimately, Just about every bug you repair provides a new layer to the talent set. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Improving your debugging expertise usually takes time, practice, and persistence — although the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.